Updated for model loader flags
Родитель
11735861c5
Коммит
f48b4f357e
|
@ -53,8 +53,8 @@ Here is an example of using the descriptor pile with model loading:
|
|||
|
||||
```cpp
|
||||
// Load models.
|
||||
car = Model::CreateFrom*(...);
|
||||
ship = Model::CreateFrom*(...);
|
||||
car = Model::CreateFrom*(device, ...);
|
||||
ship = Model::CreateFrom*(device, ...);
|
||||
|
||||
// Create sampler heap.
|
||||
states = std::make_unique<CommonStates>(device);
|
||||
|
|
12
Model.md
12
Model.md
|
@ -22,13 +22,13 @@ Model instances can be loaded from either ``.SDKMESH`` or ``.VBO`` files, or fr
|
|||
The [Samples Content Exporter](http://go.microsoft.com/fwlink/?LinkId=226208) will generate ``.SDKMESH`` files from an Autodesk ``.FBX``.
|
||||
|
||||
```cpp
|
||||
auto tiny = Model::CreateFromSDKMESH( L"tiny.sdkmesh" );
|
||||
auto tiny = Model::CreateFromSDKMESH( device, L"tiny.sdkmesh" );
|
||||
```
|
||||
|
||||
The ``.VBO`` file format is a very simple geometry format containing a index buffer and a vertex buffer. It was originally introduced in the Windows 8 ResourceLoading sample, but can be generated by DirectXMesh's [meshconvert](https://github.com/Microsoft/DirectXMesh/wiki/Meshconvert) utility.
|
||||
|
||||
```cpp
|
||||
auto ship = Model::CreateFromVBO( L"ship.vbo" );
|
||||
auto ship = Model::CreateFromVBO( device, L"ship.vbo" );
|
||||
```
|
||||
|
||||
# Materials
|
||||
|
@ -293,6 +293,14 @@ A Model instance contains a name (a wide-character string) for tracking and appl
|
|||
|
||||
The ModelMeshPart class provides a _material index_ for each submesh which describes the effect to render with. This indexes the information in Model for _materials_ which consists of [[EffectInfo|Effects]] data. The _textureNames_ is a list of the texture filenames indexed by the ``EffectInfo``.
|
||||
|
||||
# Model Loader Flags
|
||||
|
||||
The various ``CreateFrom*`` methods have a defaulted parameter to provide additional model loader controls.
|
||||
|
||||
* ``ModelLoader_MaterialColorsSRGB``: Material colors specified in the model file should be converted from sRGB to Linear colorspace.
|
||||
|
||||
* ``ModelLoader_AllowLargeModels``: Allows models with VBs/IBs that exceed the required resource size support for all Direct3D devices as indicated by the ``D3D12_REQ_RESOURCE_SIZE_IN_MEGABYTES_EXPRESSION_x_TERM`` constants.
|
||||
|
||||
# Content Notes
|
||||
|
||||
See [Geometry formats](https://github.com/Microsoft/DirectXMesh/wiki/Geometry-formats) for more information.
|
||||
|
|
|
@ -47,7 +47,7 @@ In **Game.cpp**, add to the TODO of **CreateDevice** right after you create ``m_
|
|||
```cpp
|
||||
m_states = std::make_unique<CommonStates>(m_d3dDevice.Get());
|
||||
|
||||
m_model = Model::CreateFromSDKMESH(L"cup.sdkmesh");
|
||||
m_model = Model::CreateFromSDKMESH(m_d3dDevice.Get(), L"cup.sdkmesh");
|
||||
|
||||
ResourceUploadBatch resourceUpload(m_d3dDevice.Get());
|
||||
|
||||
|
@ -126,7 +126,7 @@ Build and run and you will see our cup model rendered with default lighting:
|
|||
|
||||
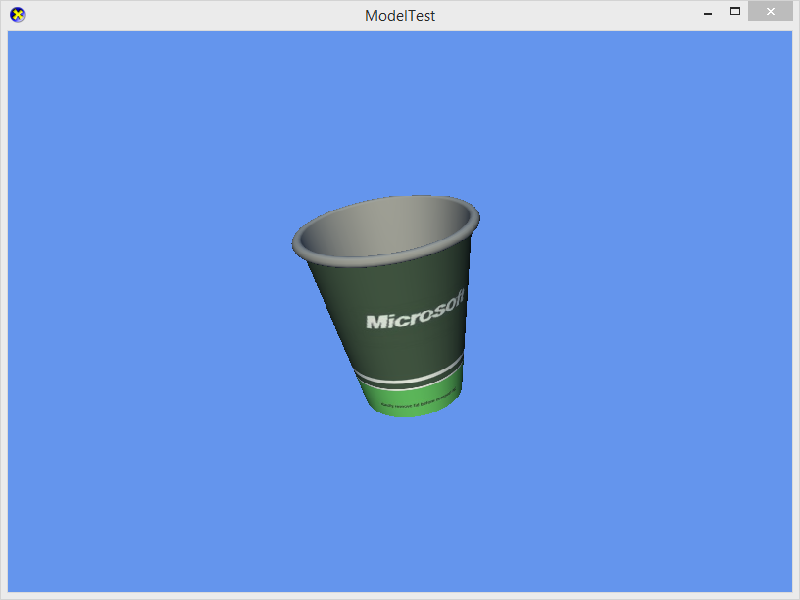
|
||||
|
||||
> _Troubleshooting:_ If you get a runtime exception, then you may have the "cup.jpg" or "cup.sdkmesh" in the wrong folder, have modified the "Working Directory" in the "Debugging" configuration settings, or otherwise changed the expected paths at runtime of the application. You should set a break-point on ``Model::CreateFromSDKMESH`` and step into the code to find the exact problem.
|
||||
> _Troubleshooting:_ If you get a runtime exception, then you may have the "cup.jpg" or "cup.sdkmesh" in the wrong folder, have modified the "Working Directory" in the "Debugging" configuration settings, or otherwise changed the expected paths at runtime of the application. You should set a break-point on ``Model::4SDKMESH`` and step into the code to find the exact problem.
|
||||
|
||||
> The render function must set descriptor heaps using ``SetDescriptorHeaps``. This allows the application to use their own heaps instead of the ``EffectTextureFactory`` class for where the textures are in memory. You can freely mix and match heaps in the application, but remember that you can have only a single texture descriptor heap and a single sampler descriptor heap active at any given time.
|
||||
|
||||
|
|
Загрузка…
Ссылка в новой задаче