2015-02-20 07:10:52 +03:00
|
|
|
/**
|
2015-03-23 23:35:08 +03:00
|
|
|
* Copyright (c) 2015-present, Facebook, Inc.
|
|
|
|
* All rights reserved.
|
|
|
|
*
|
|
|
|
* This source code is licensed under the BSD-style license found in the
|
|
|
|
* LICENSE file in the root directory of this source tree. An additional grant
|
|
|
|
* of patent rights can be found in the PATENTS file in the same directory.
|
2015-02-20 07:10:52 +03:00
|
|
|
*
|
2017-02-25 21:21:09 +03:00
|
|
|
* @providesModule react-native-implementation
|
2017-04-05 20:16:30 +03:00
|
|
|
* @flow
|
2015-02-20 07:10:52 +03:00
|
|
|
*/
|
|
|
|
'use strict';
|
|
|
|
|
2017-03-27 23:27:12 +03:00
|
|
|
const invariant = require('fbjs/lib/invariant');
|
2016-04-12 04:19:23 +03:00
|
|
|
|
2015-03-23 08:30:06 +03:00
|
|
|
// Export React, plus some native additions.
|
2016-05-25 14:17:35 +03:00
|
|
|
const ReactNative = {
|
2015-03-18 20:06:01 +03:00
|
|
|
// Components
|
2017-02-28 05:28:23 +03:00
|
|
|
get AccessibilityInfo() { return require('AccessibilityInfo'); },
|
2016-05-26 23:46:58 +03:00
|
|
|
get ActivityIndicator() { return require('ActivityIndicator'); },
|
2015-12-08 03:54:18 +03:00
|
|
|
get ART() { return require('ReactNativeART'); },
|
2016-10-11 03:18:42 +03:00
|
|
|
get Button() { return require('Button'); },
|
2017-08-25 20:22:17 +03:00
|
|
|
get CheckBox() { return require('CheckBox'); },
|
2015-12-08 03:54:18 +03:00
|
|
|
get DatePickerIOS() { return require('DatePickerIOS'); },
|
|
|
|
get DrawerLayoutAndroid() { return require('DrawerLayoutAndroid'); },
|
2017-03-02 04:52:52 +03:00
|
|
|
get FlatList() { return require('FlatList'); },
|
2015-12-08 03:54:18 +03:00
|
|
|
get Image() { return require('Image'); },
|
2017-05-24 21:19:31 +03:00
|
|
|
get ImageBackground() { return require('ImageBackground'); },
|
2015-12-23 00:36:37 +03:00
|
|
|
get ImageEditor() { return require('ImageEditor'); },
|
|
|
|
get ImageStore() { return require('ImageStore'); },
|
2016-06-07 17:42:50 +03:00
|
|
|
get KeyboardAvoidingView() { return require('KeyboardAvoidingView'); },
|
2015-12-08 03:54:18 +03:00
|
|
|
get ListView() { return require('ListView'); },
|
MaskedViewIOS -- A way to apply alpha masks to views on iOS
Summary:
It's very important in complex UIs to be able to apply alpha channel-based masks to arbitrary content. Common use cases include adding gradient masks at the top or bottom of scroll views, creating masked text effects, feathering images, and generally just masking views while still allowing transparency of those views.
The original motivation for creating this component stemmed from work on `react-navigation`. As I tried to mimic behavior in the native iOS header, I needed to be able to achieve the effect pictured here (this is a screenshot from a native iOS application):
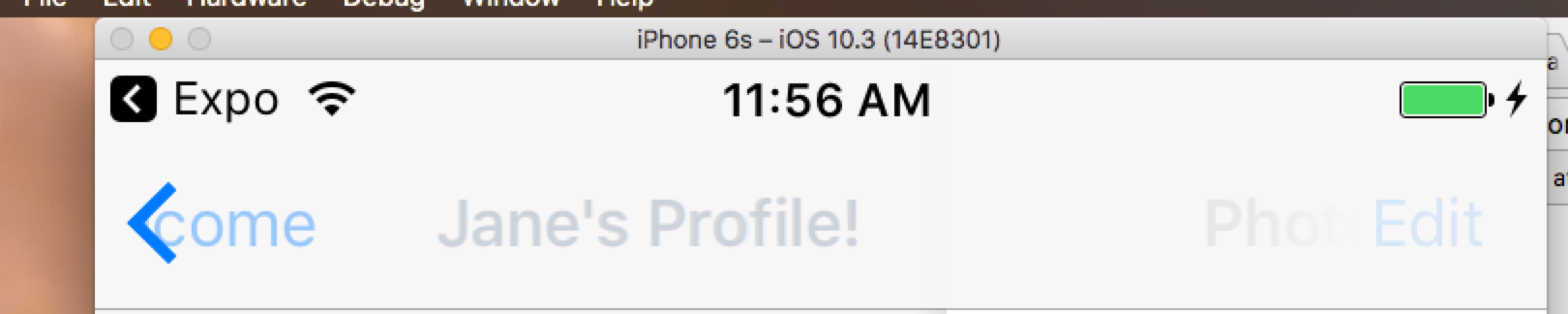
In this image, there are two masks:
- A mask on the back button chevron
- A gradient mask on the right button
In addition, the underlying view in the navigation bar is intended to be a UIBlurView. Thus, alpha masking is the only way to achieve this effect.
Behind the scenes, the `maskView` property on `UIView` is used. This is a shortcut to setting the mask on the CALayer directly.
This gives us the ability to mask any view with any other view. While building this component (and testing in the context of an Expo app), I was able to use a `GLView` (a view that renders an OpenGL context) to mask a `Video` component!
I chose to implement this only on iOS right now, as the Android implementation is a) significantly more complicated and b) will most likely not be as performant (especially when trying to mask more complex views).
Review the `<MaskedViewIOS>` section in the RNTester app, observe that views are masked appropriately.

Closes https://github.com/facebook/react-native/pull/14898
Differential Revision: D5398721
Pulled By: javache
fbshipit-source-id: 343af874e2d664541aca1fefe922cf7d82aea701
2017-07-12 01:00:54 +03:00
|
|
|
get MaskedViewIOS() { return require('MaskedViewIOS'); },
|
2015-12-08 03:54:18 +03:00
|
|
|
get Modal() { return require('Modal'); },
|
|
|
|
get NavigatorIOS() { return require('NavigatorIOS'); },
|
2016-01-29 14:58:35 +03:00
|
|
|
get Picker() { return require('Picker'); },
|
2015-12-08 03:54:18 +03:00
|
|
|
get PickerIOS() { return require('PickerIOS'); },
|
|
|
|
get ProgressBarAndroid() { return require('ProgressBarAndroid'); },
|
|
|
|
get ProgressViewIOS() { return require('ProgressViewIOS'); },
|
2017-09-25 08:57:31 +03:00
|
|
|
get SafeAreaView() { return require('SafeAreaView'); },
|
2015-12-08 03:54:18 +03:00
|
|
|
get ScrollView() { return require('ScrollView'); },
|
2017-03-02 04:52:52 +03:00
|
|
|
get SectionList() { return require('SectionList'); },
|
2015-12-08 03:54:18 +03:00
|
|
|
get SegmentedControlIOS() { return require('SegmentedControlIOS'); },
|
2016-04-06 14:49:47 +03:00
|
|
|
get Slider() { return require('Slider'); },
|
2015-12-08 03:54:18 +03:00
|
|
|
get SnapshotViewIOS() { return require('SnapshotViewIOS'); },
|
|
|
|
get Switch() { return require('Switch'); },
|
2016-01-04 18:59:10 +03:00
|
|
|
get RefreshControl() { return require('RefreshControl'); },
|
2016-02-03 17:40:39 +03:00
|
|
|
get StatusBar() { return require('StatusBar'); },
|
2017-09-29 08:14:45 +03:00
|
|
|
get SwipeableFlatList() { return require('SwipeableFlatList'); },
|
2016-07-11 14:55:59 +03:00
|
|
|
get SwipeableListView() { return require('SwipeableListView'); },
|
2015-12-08 03:54:18 +03:00
|
|
|
get TabBarIOS() { return require('TabBarIOS'); },
|
|
|
|
get Text() { return require('Text'); },
|
|
|
|
get TextInput() { return require('TextInput'); },
|
|
|
|
get ToastAndroid() { return require('ToastAndroid'); },
|
|
|
|
get ToolbarAndroid() { return require('ToolbarAndroid'); },
|
|
|
|
get Touchable() { return require('Touchable'); },
|
|
|
|
get TouchableHighlight() { return require('TouchableHighlight'); },
|
|
|
|
get TouchableNativeFeedback() { return require('TouchableNativeFeedback'); },
|
|
|
|
get TouchableOpacity() { return require('TouchableOpacity'); },
|
|
|
|
get TouchableWithoutFeedback() { return require('TouchableWithoutFeedback'); },
|
|
|
|
get View() { return require('View'); },
|
|
|
|
get ViewPagerAndroid() { return require('ViewPagerAndroid'); },
|
2017-03-02 04:52:52 +03:00
|
|
|
get VirtualizedList() { return require('VirtualizedList'); },
|
2015-12-08 03:54:18 +03:00
|
|
|
get WebView() { return require('WebView'); },
|
2015-03-18 20:06:01 +03:00
|
|
|
|
|
|
|
// APIs
|
2015-12-08 03:54:18 +03:00
|
|
|
get ActionSheetIOS() { return require('ActionSheetIOS'); },
|
2015-12-17 22:09:22 +03:00
|
|
|
get Alert() { return require('Alert'); },
|
2015-12-08 03:54:18 +03:00
|
|
|
get AlertIOS() { return require('AlertIOS'); },
|
|
|
|
get Animated() { return require('Animated'); },
|
|
|
|
get AppRegistry() { return require('AppRegistry'); },
|
2016-01-21 22:43:51 +03:00
|
|
|
get AppState() { return require('AppState'); },
|
2015-12-08 03:54:18 +03:00
|
|
|
get AsyncStorage() { return require('AsyncStorage'); },
|
2017-03-07 08:41:51 +03:00
|
|
|
get BackAndroid() { return require('BackAndroid'); }, // deprecated: use BackHandler instead
|
|
|
|
get BackHandler() { return require('BackHandler'); },
|
2015-12-08 03:54:18 +03:00
|
|
|
get CameraRoll() { return require('CameraRoll'); },
|
2015-12-09 21:00:19 +03:00
|
|
|
get Clipboard() { return require('Clipboard'); },
|
2016-01-26 21:29:47 +03:00
|
|
|
get DatePickerAndroid() { return require('DatePickerAndroid'); },
|
2017-03-18 02:47:51 +03:00
|
|
|
get DeviceInfo() { return require('DeviceInfo'); },
|
2015-12-08 03:54:18 +03:00
|
|
|
get Dimensions() { return require('Dimensions'); },
|
|
|
|
get Easing() { return require('Easing'); },
|
2017-05-02 23:37:38 +03:00
|
|
|
get findNodeHandle() { return require('ReactNative').findNodeHandle; },
|
2016-08-19 06:49:25 +03:00
|
|
|
get I18nManager() { return require('I18nManager'); },
|
2015-12-08 03:54:18 +03:00
|
|
|
get ImagePickerIOS() { return require('ImagePickerIOS'); },
|
|
|
|
get InteractionManager() { return require('InteractionManager'); },
|
2016-05-25 14:17:35 +03:00
|
|
|
get Keyboard() { return require('Keyboard'); },
|
2015-12-08 03:54:18 +03:00
|
|
|
get LayoutAnimation() { return require('LayoutAnimation'); },
|
2016-01-27 01:34:00 +03:00
|
|
|
get Linking() { return require('Linking'); },
|
2016-05-25 14:17:35 +03:00
|
|
|
get NativeEventEmitter() { return require('NativeEventEmitter'); },
|
2015-12-08 03:54:18 +03:00
|
|
|
get NetInfo() { return require('NetInfo'); },
|
|
|
|
get PanResponder() { return require('PanResponder'); },
|
2016-08-15 15:52:04 +03:00
|
|
|
get PermissionsAndroid() { return require('PermissionsAndroid'); },
|
2015-12-08 03:54:18 +03:00
|
|
|
get PixelRatio() { return require('PixelRatio'); },
|
|
|
|
get PushNotificationIOS() { return require('PushNotificationIOS'); },
|
|
|
|
get Settings() { return require('Settings'); },
|
2016-07-25 13:34:06 +03:00
|
|
|
get Share() { return require('Share'); },
|
2015-12-08 03:54:18 +03:00
|
|
|
get StatusBarIOS() { return require('StatusBarIOS'); },
|
|
|
|
get StyleSheet() { return require('StyleSheet'); },
|
2016-05-25 15:21:36 +03:00
|
|
|
get Systrace() { return require('Systrace'); },
|
2016-01-26 21:29:47 +03:00
|
|
|
get TimePickerAndroid() { return require('TimePickerAndroid'); },
|
2017-03-07 08:41:51 +03:00
|
|
|
get TVEventHandler() { return require('TVEventHandler'); },
|
2015-12-08 03:54:18 +03:00
|
|
|
get UIManager() { return require('UIManager'); },
|
2017-06-21 10:30:39 +03:00
|
|
|
get unstable_batchedUpdates() { return require('ReactNative').unstable_batchedUpdates; },
|
2016-03-03 15:08:10 +03:00
|
|
|
get Vibration() { return require('Vibration'); },
|
2015-12-08 03:54:18 +03:00
|
|
|
get VibrationIOS() { return require('VibrationIOS'); },
|
2015-03-18 20:06:01 +03:00
|
|
|
|
2015-03-23 19:39:18 +03:00
|
|
|
// Plugins
|
2015-12-08 03:54:18 +03:00
|
|
|
get DeviceEventEmitter() { return require('RCTDeviceEventEmitter'); },
|
|
|
|
get NativeAppEventEmitter() { return require('RCTNativeAppEventEmitter'); },
|
|
|
|
get NativeModules() { return require('NativeModules'); },
|
|
|
|
get Platform() { return require('Platform'); },
|
|
|
|
get processColor() { return require('processColor'); },
|
|
|
|
get requireNativeComponent() { return require('requireNativeComponent'); },
|
2017-04-05 20:16:30 +03:00
|
|
|
get takeSnapshot() { return require('takeSnapshot'); },
|
2015-03-23 19:39:18 +03:00
|
|
|
|
2015-06-12 21:14:19 +03:00
|
|
|
// Prop Types
|
2015-12-23 06:29:01 +03:00
|
|
|
get ColorPropType() { return require('ColorPropType'); },
|
2015-12-08 03:54:18 +03:00
|
|
|
get EdgeInsetsPropType() { return require('EdgeInsetsPropType'); },
|
|
|
|
get PointPropType() { return require('PointPropType'); },
|
2017-03-24 03:31:16 +03:00
|
|
|
get ViewPropTypes() { return require('ViewPropTypes'); },
|
2017-03-27 23:27:12 +03:00
|
|
|
|
|
|
|
// Deprecated
|
|
|
|
get Navigator() {
|
|
|
|
invariant(
|
|
|
|
false,
|
|
|
|
'Navigator is deprecated and has been removed from this package. It can now be installed ' +
|
|
|
|
'and imported from `react-native-deprecated-custom-components` instead of `react-native`. ' +
|
|
|
|
'Learn about alternative navigation solutions at http://facebook.github.io/react-native/docs/navigation.html'
|
|
|
|
);
|
|
|
|
},
|
2015-12-08 03:54:18 +03:00
|
|
|
};
|
2015-02-20 07:10:52 +03:00
|
|
|
|
|
|
|
module.exports = ReactNative;
|