2019-10-16 20:03:47 +03:00
|
|
|
/*
|
2021-12-31 02:08:43 +03:00
|
|
|
* Copyright (c) Meta Platforms, Inc. and affiliates.
|
2015-03-23 23:28:42 +03:00
|
|
|
*
|
2018-02-17 05:24:55 +03:00
|
|
|
* This source code is licensed under the MIT license found in the
|
|
|
|
* LICENSE file in the root directory of this source tree.
|
2015-03-23 23:28:42 +03:00
|
|
|
*/
|
2015-02-20 07:10:52 +03:00
|
|
|
|
|
|
|
#import "RCTViewManager.h"
|
|
|
|
|
2021-05-04 07:29:56 +03:00
|
|
|
#import "RCTAssert.h"
|
2022-07-21 14:11:30 +03:00
|
|
|
#import "RCTBorderCurve.h"
|
2015-12-01 18:41:20 +03:00
|
|
|
#import "RCTBorderStyle.h"
|
2016-11-23 18:47:52 +03:00
|
|
|
#import "RCTBridge.h"
|
2020-03-09 08:58:01 +03:00
|
|
|
#import "RCTConvert+Transform.h"
|
2015-02-20 07:10:52 +03:00
|
|
|
#import "RCTConvert.h"
|
|
|
|
#import "RCTLog.h"
|
|
|
|
#import "RCTShadowView.h"
|
2015-04-18 20:43:20 +03:00
|
|
|
#import "RCTUIManager.h"
|
2017-09-26 23:55:58 +03:00
|
|
|
#import "RCTUIManagerUtils.h"
|
2015-03-02 02:33:55 +03:00
|
|
|
#import "RCTUtils.h"
|
2015-02-20 07:10:52 +03:00
|
|
|
#import "RCTView.h"
|
2015-05-19 16:21:52 +03:00
|
|
|
#import "UIView+React.h"
|
2015-02-20 07:10:52 +03:00
|
|
|
|
2020-03-09 08:58:01 +03:00
|
|
|
@implementation RCTConvert (UIAccessibilityTraits)
|
|
|
|
|
|
|
|
RCT_MULTI_ENUM_CONVERTER(
|
|
|
|
UIAccessibilityTraits,
|
|
|
|
(@{
|
|
|
|
@"none" : @(UIAccessibilityTraitNone),
|
|
|
|
@"button" : @(UIAccessibilityTraitButton),
|
2021-05-03 21:48:33 +03:00
|
|
|
@"togglebutton" : @(UIAccessibilityTraitButton),
|
2020-03-09 08:58:01 +03:00
|
|
|
@"link" : @(UIAccessibilityTraitLink),
|
|
|
|
@"header" : @(UIAccessibilityTraitHeader),
|
|
|
|
@"search" : @(UIAccessibilityTraitSearchField),
|
|
|
|
@"image" : @(UIAccessibilityTraitImage),
|
|
|
|
@"imagebutton" : @(UIAccessibilityTraitImage | UIAccessibilityTraitButton),
|
|
|
|
@"selected" : @(UIAccessibilityTraitSelected),
|
|
|
|
@"plays" : @(UIAccessibilityTraitPlaysSound),
|
|
|
|
@"key" : @(UIAccessibilityTraitKeyboardKey),
|
|
|
|
@"keyboardkey" : @(UIAccessibilityTraitKeyboardKey),
|
|
|
|
@"text" : @(UIAccessibilityTraitStaticText),
|
|
|
|
@"summary" : @(UIAccessibilityTraitSummaryElement),
|
|
|
|
@"disabled" : @(UIAccessibilityTraitNotEnabled),
|
|
|
|
@"frequentUpdates" : @(UIAccessibilityTraitUpdatesFrequently),
|
|
|
|
@"startsMedia" : @(UIAccessibilityTraitStartsMediaSession),
|
|
|
|
@"adjustable" : @(UIAccessibilityTraitAdjustable),
|
|
|
|
@"allowsDirectInteraction" : @(UIAccessibilityTraitAllowsDirectInteraction),
|
|
|
|
@"pageTurn" : @(UIAccessibilityTraitCausesPageTurn),
|
|
|
|
@"alert" : @(UIAccessibilityTraitNone),
|
|
|
|
@"checkbox" : @(UIAccessibilityTraitNone),
|
|
|
|
@"combobox" : @(UIAccessibilityTraitNone),
|
|
|
|
@"menu" : @(UIAccessibilityTraitNone),
|
|
|
|
@"menubar" : @(UIAccessibilityTraitNone),
|
|
|
|
@"menuitem" : @(UIAccessibilityTraitNone),
|
2021-08-25 15:09:10 +03:00
|
|
|
@"progressbar" : @(UIAccessibilityTraitUpdatesFrequently),
|
2020-03-09 08:58:01 +03:00
|
|
|
@"radio" : @(UIAccessibilityTraitNone),
|
|
|
|
@"radiogroup" : @(UIAccessibilityTraitNone),
|
|
|
|
@"scrollbar" : @(UIAccessibilityTraitNone),
|
|
|
|
@"spinbutton" : @(UIAccessibilityTraitNone),
|
|
|
|
@"switch" : @(SwitchAccessibilityTrait),
|
|
|
|
@"tab" : @(UIAccessibilityTraitNone),
|
2021-08-24 12:29:39 +03:00
|
|
|
@"tabbar" : @(UIAccessibilityTraitTabBar),
|
2020-03-09 08:58:01 +03:00
|
|
|
@"tablist" : @(UIAccessibilityTraitNone),
|
|
|
|
@"timer" : @(UIAccessibilityTraitNone),
|
|
|
|
@"toolbar" : @(UIAccessibilityTraitNone),
|
2021-07-10 04:26:23 +03:00
|
|
|
@"list" : @(UIAccessibilityTraitNone),
|
2020-03-09 08:58:01 +03:00
|
|
|
}),
|
|
|
|
UIAccessibilityTraitNone,
|
|
|
|
unsignedLongLongValue)
|
2015-05-18 17:32:21 +03:00
|
|
|
|
|
|
|
@end
|
|
|
|
|
2015-02-20 07:10:52 +03:00
|
|
|
@implementation RCTViewManager
|
|
|
|
|
2015-04-08 15:42:43 +03:00
|
|
|
@synthesize bridge = _bridge;
|
2015-02-20 07:10:52 +03:00
|
|
|
|
2015-04-08 18:52:48 +03:00
|
|
|
RCT_EXPORT_MODULE()
|
|
|
|
|
2015-04-18 20:43:20 +03:00
|
|
|
- (dispatch_queue_t)methodQueue
|
|
|
|
{
|
2016-05-16 18:01:35 +03:00
|
|
|
return RCTGetUIManagerQueue();
|
2015-04-18 20:43:20 +03:00
|
|
|
}
|
|
|
|
|
2021-05-04 07:29:56 +03:00
|
|
|
- (void)setBridge:(RCTBridge *)bridge
|
|
|
|
{
|
2022-03-23 06:14:32 +03:00
|
|
|
RCTErrorNewArchitectureValidation(
|
|
|
|
RCTNotAllowedInBridgeless, self, @"RCTViewManager must not be initialized for the new architecture");
|
2021-05-04 07:29:56 +03:00
|
|
|
_bridge = bridge;
|
|
|
|
}
|
|
|
|
|
2015-02-20 07:10:52 +03:00
|
|
|
- (UIView *)view
|
|
|
|
{
|
2015-08-17 17:35:34 +03:00
|
|
|
return [RCTView new];
|
2015-02-20 07:10:52 +03:00
|
|
|
}
|
|
|
|
|
|
|
|
- (RCTShadowView *)shadowView
|
|
|
|
{
|
2015-08-17 17:35:34 +03:00
|
|
|
return [RCTShadowView new];
|
2015-02-20 07:10:52 +03:00
|
|
|
}
|
|
|
|
|
2015-11-04 01:45:46 +03:00
|
|
|
- (NSArray<NSString *> *)customBubblingEventTypes
|
2015-02-20 07:10:52 +03:00
|
|
|
{
|
2015-08-11 16:37:12 +03:00
|
|
|
return @[
|
|
|
|
|
|
|
|
// Generic events
|
|
|
|
@"press",
|
|
|
|
@"change",
|
|
|
|
@"focus",
|
|
|
|
@"blur",
|
|
|
|
@"submitEditing",
|
|
|
|
@"endEditing",
|
2015-11-02 20:13:41 +03:00
|
|
|
@"keyPress",
|
2015-08-11 16:37:12 +03:00
|
|
|
|
|
|
|
// Touch events
|
|
|
|
@"touchStart",
|
|
|
|
@"touchMove",
|
|
|
|
@"touchCancel",
|
|
|
|
@"touchEnd",
|
|
|
|
];
|
2015-02-20 07:10:52 +03:00
|
|
|
}
|
|
|
|
|
2015-03-17 17:04:39 +03:00
|
|
|
#pragma mark - View properties
|
2015-02-20 07:10:52 +03:00
|
|
|
|
2019-07-23 13:19:41 +03:00
|
|
|
// Accessibility related properties
|
2017-06-03 00:17:56 +03:00
|
|
|
RCT_REMAP_VIEW_PROPERTY(accessible, reactAccessibilityElement.isAccessibilityElement, BOOL)
|
2019-05-20 11:24:35 +03:00
|
|
|
RCT_REMAP_VIEW_PROPERTY(accessibilityActions, reactAccessibilityElement.accessibilityActions, NSDictionaryArray)
|
2017-06-03 00:17:56 +03:00
|
|
|
RCT_REMAP_VIEW_PROPERTY(accessibilityLabel, reactAccessibilityElement.accessibilityLabel, NSString)
|
2018-07-26 03:33:58 +03:00
|
|
|
RCT_REMAP_VIEW_PROPERTY(accessibilityHint, reactAccessibilityElement.accessibilityHint, NSString)
|
2022-03-07 20:43:30 +03:00
|
|
|
RCT_REMAP_VIEW_PROPERTY(accessibilityLanguage, reactAccessibilityElement.accessibilityLanguage, NSString)
|
2019-09-18 13:14:39 +03:00
|
|
|
RCT_REMAP_VIEW_PROPERTY(accessibilityValue, reactAccessibilityElement.accessibilityValueInternal, NSDictionary)
|
2017-06-03 00:17:56 +03:00
|
|
|
RCT_REMAP_VIEW_PROPERTY(accessibilityViewIsModal, reactAccessibilityElement.accessibilityViewIsModal, BOOL)
|
2018-01-30 01:20:06 +03:00
|
|
|
RCT_REMAP_VIEW_PROPERTY(accessibilityElementsHidden, reactAccessibilityElement.accessibilityElementsHidden, BOOL)
|
2020-03-09 08:58:01 +03:00
|
|
|
RCT_REMAP_VIEW_PROPERTY(
|
|
|
|
accessibilityIgnoresInvertColors,
|
|
|
|
reactAccessibilityElement.shouldAccessibilityIgnoresInvertColors,
|
|
|
|
BOOL)
|
2017-12-05 10:19:47 +03:00
|
|
|
RCT_REMAP_VIEW_PROPERTY(onAccessibilityAction, reactAccessibilityElement.onAccessibilityAction, RCTDirectEventBlock)
|
2017-06-03 00:17:56 +03:00
|
|
|
RCT_REMAP_VIEW_PROPERTY(onAccessibilityTap, reactAccessibilityElement.onAccessibilityTap, RCTDirectEventBlock)
|
|
|
|
RCT_REMAP_VIEW_PROPERTY(onMagicTap, reactAccessibilityElement.onMagicTap, RCTDirectEventBlock)
|
2018-12-07 06:39:42 +03:00
|
|
|
RCT_REMAP_VIEW_PROPERTY(onAccessibilityEscape, reactAccessibilityElement.onAccessibilityEscape, RCTDirectEventBlock)
|
2017-06-03 00:17:56 +03:00
|
|
|
RCT_REMAP_VIEW_PROPERTY(testID, reactAccessibilityElement.accessibilityIdentifier, NSString)
|
|
|
|
|
2015-03-26 07:29:28 +03:00
|
|
|
RCT_EXPORT_VIEW_PROPERTY(backgroundColor, UIColor)
|
2015-07-15 03:03:41 +03:00
|
|
|
RCT_REMAP_VIEW_PROPERTY(backfaceVisibility, layer.doubleSided, css_backface_visibility_t)
|
2015-03-26 07:29:28 +03:00
|
|
|
RCT_REMAP_VIEW_PROPERTY(opacity, alpha, CGFloat)
|
2016-06-07 10:08:16 +03:00
|
|
|
RCT_REMAP_VIEW_PROPERTY(shadowColor, layer.shadowColor, CGColor)
|
|
|
|
RCT_REMAP_VIEW_PROPERTY(shadowOffset, layer.shadowOffset, CGSize)
|
2015-04-01 03:34:51 +03:00
|
|
|
RCT_REMAP_VIEW_PROPERTY(shadowOpacity, layer.shadowOpacity, float)
|
2015-03-26 07:29:28 +03:00
|
|
|
RCT_REMAP_VIEW_PROPERTY(shadowRadius, layer.shadowRadius, CGFloat)
|
2019-02-14 03:24:41 +03:00
|
|
|
RCT_REMAP_VIEW_PROPERTY(needsOffscreenAlphaCompositing, layer.allowsGroupOpacity, BOOL)
|
2016-12-02 16:47:43 +03:00
|
|
|
RCT_CUSTOM_VIEW_PROPERTY(overflow, YGOverflow, RCTView)
|
2016-08-30 10:58:15 +03:00
|
|
|
{
|
|
|
|
if (json) {
|
2016-12-02 16:47:43 +03:00
|
|
|
view.clipsToBounds = [RCTConvert YGOverflow:json] != YGOverflowVisible;
|
2016-08-30 10:58:15 +03:00
|
|
|
} else {
|
|
|
|
view.clipsToBounds = defaultView.clipsToBounds;
|
|
|
|
}
|
|
|
|
}
|
2016-11-19 01:33:23 +03:00
|
|
|
RCT_CUSTOM_VIEW_PROPERTY(shouldRasterizeIOS, BOOL, RCTView)
|
2015-08-05 02:37:38 +03:00
|
|
|
{
|
|
|
|
view.layer.shouldRasterize = json ? [RCTConvert BOOL:json] : defaultView.layer.shouldRasterize;
|
2020-03-09 08:58:01 +03:00
|
|
|
view.layer.rasterizationScale =
|
|
|
|
view.layer.shouldRasterize ? [UIScreen mainScreen].scale : defaultView.layer.rasterizationScale;
|
2015-08-05 02:37:38 +03:00
|
|
|
}
|
2017-02-08 00:59:17 +03:00
|
|
|
|
2016-11-19 01:33:23 +03:00
|
|
|
RCT_CUSTOM_VIEW_PROPERTY(transform, CATransform3D, RCTView)
|
2016-04-30 00:17:58 +03:00
|
|
|
{
|
|
|
|
view.layer.transform = json ? [RCTConvert CATransform3D:json] : defaultView.layer.transform;
|
Add allowsEdgeAntialiasing on views with rotations or skew tr… (#32920)
Summary:
…ansforms
On iOS, if a View is rotated with the a transform (e.g. <View style={{transform: {rotationZ: 5}}} />), the view has aliasing (see screenshot). Same for a skew transformation. We don't have the issue on Android
This behavior had originally being fixed by this PR https://github.com/facebook/react-native/pull/1999
However a new PR was merge ( https://github.com/facebook/react-native/pull/19360 ) that broke this. I think it was made to add antialiasing during perspective transforms but seems to have broken the antialiasing when rotationZ transforms
This PR adds back the antialising during rotation transform , while keeping it during perspective transform.
## Changelog
I changed the allowsEdgeAntialiasing condition, making it "true" when the m12 or m21 is not 0. From this article https://medium.com/swlh/understanding-3d-matrix-transforms-with-pixijs-c76da3f8bd8 , I've understood that in all rotation or skew transformations, m12 or m21 is different than 0 . In the other transformation (e.g. scale or translate) it stays at 0.
Although, I'm not a matrix transformation expert so I may be mistaken
Pull Request resolved: https://github.com/facebook/react-native/pull/32920
Test Plan:
I've written several views with all rotateX/Y/Z , skewX,Y and perpective transformation. Before the PR some transformation was showing aliasing on iOS (e.g. top-left view in the screenshot, don't hesitate to zoom in the image if you don't see it) and with this PR it does not have anymore
Before
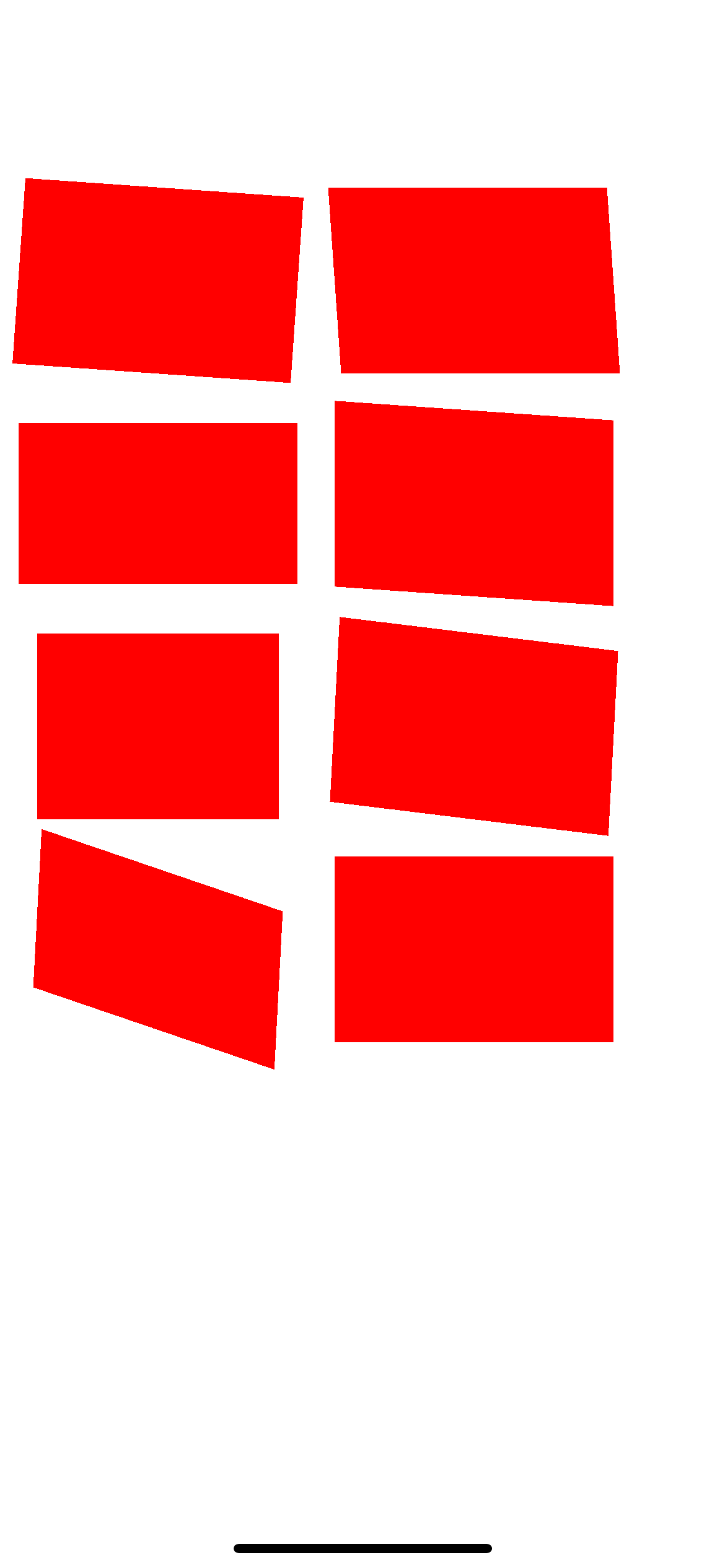
After
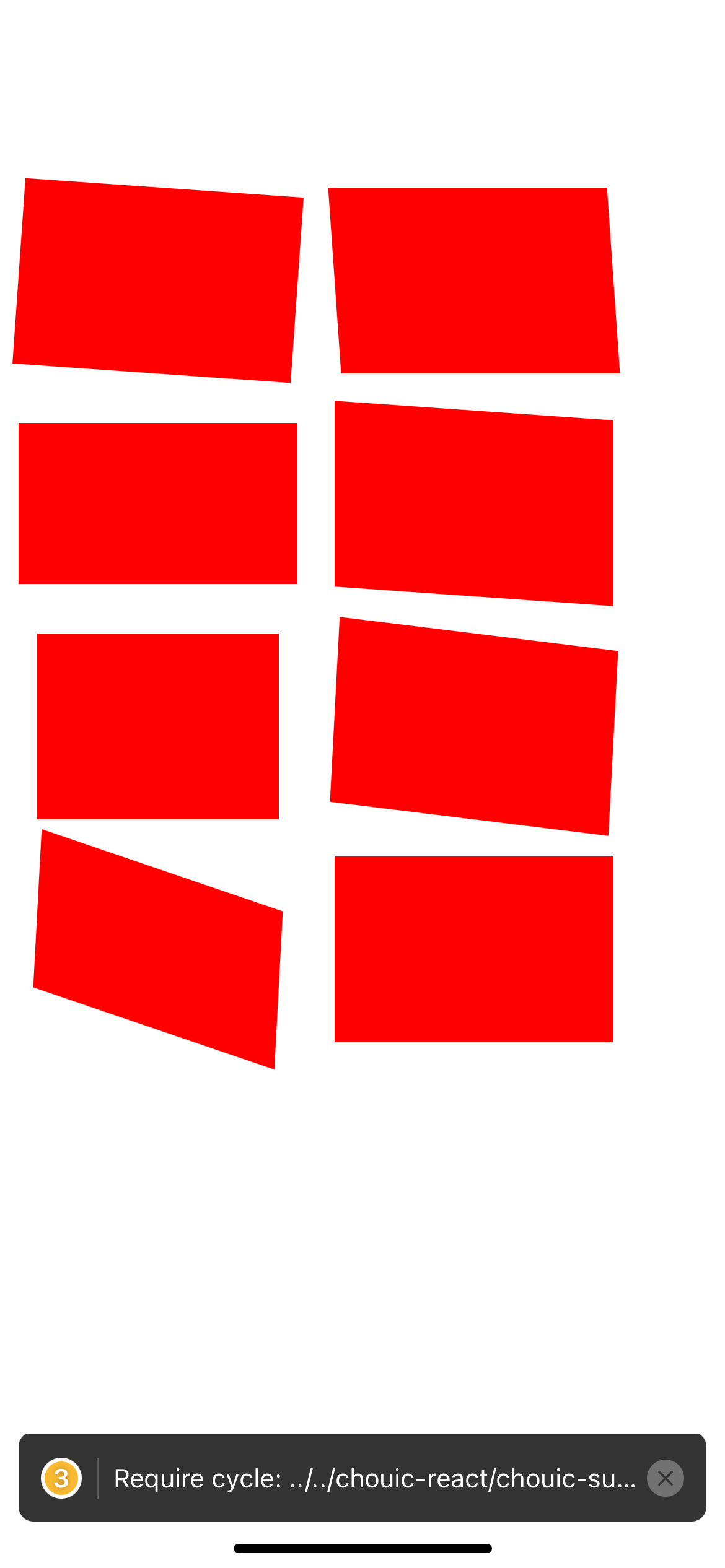
Code I used to test
```
const commonStyle = {
width: 150,
height: 100,
backgroundColor: "red",
margin: 10,
}
const Test = () => (
<View style={{ flexDirection: "row" }}>
<View>
<View
style={[
commonStyle,
{
transform: [{ rotateZ: "4deg" }],
},
]}
/>
<View
style={[
commonStyle,
{
transform: [{ rotateX: "30deg" }],
},
]}
/>
<View
style={[
commonStyle,
{
transform: [{ rotateY: "30deg" }],
},
]}
/>
<View
style={[
commonStyle,
{
transform: [{ rotateX: "30deg" }, { rotateY: "30deg" }, { rotateZ: "3deg" }],
},
]}
/>
</View>
<View>
<View
style={[
commonStyle,
{
transform: [{ skewX: "4deg" }],
},
]}
/>
<View
style={[
commonStyle,
{
transform: [{ skewY: "4deg" }],
},
]}
/>
<View
style={[
commonStyle,
{
transform: [{ skewY: "4deg" }, { rotateZ: "3deg" }],
},
]}
/>
<View
style={[
commonStyle,
{
transform: [{ perspective: 1000 }],
},
]}
/>
</View>
</View>
)
```
Reviewed By: lunaleaps
Differential Revision: D33665910
Pulled By: sshic
fbshipit-source-id: 91163ec2a0897a73ddf0310d86afacea04b89bc7
2022-01-24 18:20:32 +03:00
|
|
|
// Enable edge antialiasing in rotation, skew, or perspective transforms
|
2022-01-25 14:27:31 +03:00
|
|
|
view.layer.allowsEdgeAntialiasing =
|
|
|
|
view.layer.transform.m12 != 0.0f || view.layer.transform.m21 != 0.0f || view.layer.transform.m34 != 0.0f;
|
2016-04-30 00:17:58 +03:00
|
|
|
}
|
2017-02-08 00:59:17 +03:00
|
|
|
|
2018-07-18 01:58:24 +03:00
|
|
|
RCT_CUSTOM_VIEW_PROPERTY(accessibilityRole, UIAccessibilityTraits, RCTView)
|
|
|
|
{
|
2020-03-09 08:58:01 +03:00
|
|
|
const UIAccessibilityTraits AccessibilityRolesMask = UIAccessibilityTraitNone | UIAccessibilityTraitButton |
|
|
|
|
UIAccessibilityTraitLink | UIAccessibilityTraitSearchField | UIAccessibilityTraitImage |
|
|
|
|
UIAccessibilityTraitKeyboardKey | UIAccessibilityTraitStaticText | UIAccessibilityTraitAdjustable |
|
2021-08-25 15:09:10 +03:00
|
|
|
UIAccessibilityTraitHeader | UIAccessibilityTraitSummaryElement | UIAccessibilityTraitTabBar |
|
|
|
|
UIAccessibilityTraitUpdatesFrequently | SwitchAccessibilityTrait;
|
2020-03-09 08:58:01 +03:00
|
|
|
view.reactAccessibilityElement.accessibilityTraits =
|
|
|
|
view.reactAccessibilityElement.accessibilityTraits & ~AccessibilityRolesMask;
|
2018-09-06 03:18:36 +03:00
|
|
|
UIAccessibilityTraits newTraits = json ? [RCTConvert UIAccessibilityTraits:json] : defaultView.accessibilityTraits;
|
2019-04-25 16:09:35 +03:00
|
|
|
if (newTraits != UIAccessibilityTraitNone) {
|
|
|
|
UIAccessibilityTraits maskedTraits = newTraits & AccessibilityRolesMask;
|
|
|
|
view.reactAccessibilityElement.accessibilityTraits |= maskedTraits;
|
|
|
|
} else {
|
|
|
|
NSString *role = json ? [RCTConvert NSString:json] : @"";
|
2019-05-08 13:44:40 +03:00
|
|
|
view.reactAccessibilityElement.accessibilityRole = role;
|
2019-04-25 16:09:35 +03:00
|
|
|
}
|
|
|
|
}
|
|
|
|
|
2019-05-23 15:27:39 +03:00
|
|
|
RCT_CUSTOM_VIEW_PROPERTY(accessibilityState, NSDictionary, RCTView)
|
|
|
|
{
|
|
|
|
NSDictionary<NSString *, id> *state = json ? [RCTConvert NSDictionary:json] : nil;
|
2021-10-21 08:16:48 +03:00
|
|
|
NSMutableDictionary<NSString *, id> *newState = [NSMutableDictionary<NSString *, id> new];
|
2019-05-23 15:27:39 +03:00
|
|
|
|
|
|
|
if (!state) {
|
|
|
|
return;
|
|
|
|
}
|
|
|
|
|
|
|
|
const UIAccessibilityTraits AccessibilityStatesMask = UIAccessibilityTraitNotEnabled | UIAccessibilityTraitSelected;
|
2020-03-09 08:58:01 +03:00
|
|
|
view.reactAccessibilityElement.accessibilityTraits =
|
|
|
|
view.reactAccessibilityElement.accessibilityTraits & ~AccessibilityStatesMask;
|
2019-05-23 15:27:39 +03:00
|
|
|
|
|
|
|
for (NSString *s in state) {
|
|
|
|
id val = [state objectForKey:s];
|
|
|
|
if (!val) {
|
|
|
|
continue;
|
|
|
|
}
|
|
|
|
if ([s isEqualToString:@"selected"] && [val isKindOfClass:[NSNumber class]] && [val boolValue]) {
|
|
|
|
view.reactAccessibilityElement.accessibilityTraits |= UIAccessibilityTraitSelected;
|
|
|
|
} else if ([s isEqualToString:@"disabled"] && [val isKindOfClass:[NSNumber class]] && [val boolValue]) {
|
|
|
|
view.reactAccessibilityElement.accessibilityTraits |= UIAccessibilityTraitNotEnabled;
|
|
|
|
} else {
|
|
|
|
newState[s] = val;
|
|
|
|
}
|
|
|
|
}
|
|
|
|
if (newState.count > 0) {
|
|
|
|
view.reactAccessibilityElement.accessibilityState = newState;
|
2019-10-14 10:26:41 +03:00
|
|
|
// Post a layout change notification to make sure VoiceOver get notified for the state
|
|
|
|
// changes that don't happen upon users' click.
|
|
|
|
UIAccessibilityPostNotification(UIAccessibilityLayoutChangedNotification, nil);
|
2019-05-23 15:27:39 +03:00
|
|
|
} else {
|
|
|
|
view.reactAccessibilityElement.accessibilityState = nil;
|
|
|
|
}
|
|
|
|
}
|
|
|
|
|
2019-03-05 20:37:47 +03:00
|
|
|
RCT_CUSTOM_VIEW_PROPERTY(nativeID, NSString *, RCTView)
|
|
|
|
{
|
|
|
|
view.nativeID = json ? [RCTConvert NSString:json] : defaultView.nativeID;
|
|
|
|
[_bridge.uiManager setNativeID:view.nativeID forView:view];
|
|
|
|
}
|
|
|
|
|
2015-03-26 07:29:28 +03:00
|
|
|
RCT_CUSTOM_VIEW_PROPERTY(pointerEvents, RCTPointerEvents, RCTView)
|
2015-02-20 07:10:52 +03:00
|
|
|
{
|
|
|
|
if ([view respondsToSelector:@selector(setPointerEvents:)]) {
|
|
|
|
view.pointerEvents = json ? [RCTConvert RCTPointerEvents:json] : defaultView.pointerEvents;
|
|
|
|
return;
|
|
|
|
}
|
2015-03-02 02:33:55 +03:00
|
|
|
|
2015-02-20 07:10:52 +03:00
|
|
|
if (!json) {
|
|
|
|
view.userInteractionEnabled = defaultView.userInteractionEnabled;
|
|
|
|
return;
|
|
|
|
}
|
2015-03-02 02:33:55 +03:00
|
|
|
|
2015-03-25 03:37:03 +03:00
|
|
|
switch ([RCTConvert RCTPointerEvents:json]) {
|
2015-02-20 07:10:52 +03:00
|
|
|
case RCTPointerEventsUnspecified:
|
|
|
|
// Pointer events "unspecified" acts as if a stylesheet had not specified,
|
|
|
|
// which is different than "auto" in CSS (which cannot and will not be
|
2015-03-26 12:58:06 +03:00
|
|
|
// supported in `React`. "auto" may override a parent's "none".
|
2015-02-20 07:10:52 +03:00
|
|
|
// Unspecified values do not.
|
|
|
|
// This wouldn't override a container view's `userInteractionEnabled = NO`
|
|
|
|
view.userInteractionEnabled = YES;
|
|
|
|
case RCTPointerEventsNone:
|
|
|
|
view.userInteractionEnabled = NO;
|
|
|
|
break;
|
|
|
|
default:
|
|
|
|
RCTLogError(@"UIView base class does not support pointerEvent value: %@", json);
|
|
|
|
}
|
|
|
|
}
|
2016-11-19 01:33:23 +03:00
|
|
|
RCT_CUSTOM_VIEW_PROPERTY(removeClippedSubviews, BOOL, RCTView)
|
|
|
|
{
|
|
|
|
if ([view respondsToSelector:@selector(setRemoveClippedSubviews:)]) {
|
|
|
|
view.removeClippedSubviews = json ? [RCTConvert BOOL:json] : defaultView.removeClippedSubviews;
|
|
|
|
}
|
|
|
|
}
|
2022-07-21 14:11:30 +03:00
|
|
|
RCT_CUSTOM_VIEW_PROPERTY(borderCurve, RCTBorderCurve, RCTView)
|
|
|
|
{
|
|
|
|
if (@available(iOS 13.0, *)) {
|
|
|
|
switch ([RCTConvert RCTBorderCurve:json]) {
|
|
|
|
case RCTBorderCurveContinuous:
|
|
|
|
view.layer.cornerCurve = kCACornerCurveContinuous;
|
|
|
|
break;
|
|
|
|
case RCTBorderCurveCircular:
|
|
|
|
view.layer.cornerCurve = kCACornerCurveCircular;
|
|
|
|
break;
|
|
|
|
}
|
|
|
|
}
|
|
|
|
}
|
2020-03-09 08:58:01 +03:00
|
|
|
RCT_CUSTOM_VIEW_PROPERTY(borderRadius, CGFloat, RCTView)
|
|
|
|
{
|
2015-05-13 18:22:21 +03:00
|
|
|
if ([view respondsToSelector:@selector(setBorderRadius:)]) {
|
2015-07-31 21:23:29 +03:00
|
|
|
view.borderRadius = json ? [RCTConvert CGFloat:json] : defaultView.borderRadius;
|
2015-05-13 18:22:21 +03:00
|
|
|
} else {
|
2015-07-31 21:23:29 +03:00
|
|
|
view.layer.cornerRadius = json ? [RCTConvert CGFloat:json] : defaultView.layer.cornerRadius;
|
2015-05-13 18:22:21 +03:00
|
|
|
}
|
|
|
|
}
|
2022-02-25 19:59:09 +03:00
|
|
|
RCT_CUSTOM_VIEW_PROPERTY(borderColor, UIColor, RCTView)
|
2015-03-26 11:43:17 +03:00
|
|
|
{
|
|
|
|
if ([view respondsToSelector:@selector(setBorderColor:)]) {
|
2021-06-23 23:36:11 +03:00
|
|
|
view.borderColor = json ? [RCTConvert UIColor:json] : defaultView.borderColor;
|
2015-03-26 11:43:17 +03:00
|
|
|
} else {
|
|
|
|
view.layer.borderColor = json ? [RCTConvert CGColor:json] : defaultView.layer.borderColor;
|
|
|
|
}
|
|
|
|
}
|
2016-10-26 23:12:26 +03:00
|
|
|
RCT_CUSTOM_VIEW_PROPERTY(borderWidth, float, RCTView)
|
2015-03-26 11:43:17 +03:00
|
|
|
{
|
|
|
|
if ([view respondsToSelector:@selector(setBorderWidth:)]) {
|
|
|
|
view.borderWidth = json ? [RCTConvert CGFloat:json] : defaultView.borderWidth;
|
|
|
|
} else {
|
|
|
|
view.layer.borderWidth = json ? [RCTConvert CGFloat:json] : defaultView.layer.borderWidth;
|
|
|
|
}
|
|
|
|
}
|
2015-12-01 18:41:20 +03:00
|
|
|
RCT_CUSTOM_VIEW_PROPERTY(borderStyle, RCTBorderStyle, RCTView)
|
|
|
|
{
|
|
|
|
if ([view respondsToSelector:@selector(setBorderStyle:)]) {
|
|
|
|
view.borderStyle = json ? [RCTConvert RCTBorderStyle:json] : defaultView.borderStyle;
|
|
|
|
}
|
|
|
|
}
|
2016-02-17 03:50:35 +03:00
|
|
|
RCT_CUSTOM_VIEW_PROPERTY(hitSlop, UIEdgeInsets, RCTView)
|
|
|
|
{
|
|
|
|
if ([view respondsToSelector:@selector(setHitTestEdgeInsets:)]) {
|
|
|
|
if (json) {
|
|
|
|
UIEdgeInsets hitSlopInsets = [RCTConvert UIEdgeInsets:json];
|
2020-03-09 08:58:01 +03:00
|
|
|
view.hitTestEdgeInsets =
|
|
|
|
UIEdgeInsetsMake(-hitSlopInsets.top, -hitSlopInsets.left, -hitSlopInsets.bottom, -hitSlopInsets.right);
|
2016-02-17 03:50:35 +03:00
|
|
|
} else {
|
|
|
|
view.hitTestEdgeInsets = defaultView.hitTestEdgeInsets;
|
|
|
|
}
|
|
|
|
}
|
|
|
|
}
|
2015-03-26 11:43:17 +03:00
|
|
|
|
2021-05-25 22:50:53 +03:00
|
|
|
RCT_CUSTOM_VIEW_PROPERTY(collapsable, BOOL, RCTView)
|
|
|
|
{
|
|
|
|
// Property is only to be used in the new renderer.
|
|
|
|
// It is necessary to add it here, otherwise it gets
|
|
|
|
// filtered by view configs.
|
|
|
|
}
|
|
|
|
|
2020-03-09 08:58:01 +03:00
|
|
|
#define RCT_VIEW_BORDER_PROPERTY(SIDE) \
|
|
|
|
RCT_CUSTOM_VIEW_PROPERTY(border##SIDE##Width, float, RCTView) \
|
|
|
|
{ \
|
|
|
|
if ([view respondsToSelector:@selector(setBorder##SIDE##Width:)]) { \
|
|
|
|
view.border##SIDE##Width = json ? [RCTConvert CGFloat:json] : defaultView.border##SIDE##Width; \
|
|
|
|
} \
|
|
|
|
} \
|
|
|
|
RCT_CUSTOM_VIEW_PROPERTY(border##SIDE##Color, UIColor, RCTView) \
|
|
|
|
{ \
|
|
|
|
if ([view respondsToSelector:@selector(setBorder##SIDE##Color:)]) { \
|
2021-06-23 23:36:11 +03:00
|
|
|
view.border##SIDE##Color = json ? [RCTConvert UIColor:json] : defaultView.border##SIDE##Color; \
|
2020-03-09 08:58:01 +03:00
|
|
|
} \
|
|
|
|
}
|
2015-03-26 11:43:17 +03:00
|
|
|
|
|
|
|
RCT_VIEW_BORDER_PROPERTY(Top)
|
|
|
|
RCT_VIEW_BORDER_PROPERTY(Right)
|
|
|
|
RCT_VIEW_BORDER_PROPERTY(Bottom)
|
|
|
|
RCT_VIEW_BORDER_PROPERTY(Left)
|
2017-10-19 05:29:42 +03:00
|
|
|
RCT_VIEW_BORDER_PROPERTY(Start)
|
|
|
|
RCT_VIEW_BORDER_PROPERTY(End)
|
2015-02-20 07:10:52 +03:00
|
|
|
|
2020-03-09 08:58:01 +03:00
|
|
|
#define RCT_VIEW_BORDER_RADIUS_PROPERTY(SIDE) \
|
|
|
|
RCT_CUSTOM_VIEW_PROPERTY(border##SIDE##Radius, CGFloat, RCTView) \
|
|
|
|
{ \
|
|
|
|
if ([view respondsToSelector:@selector(setBorder##SIDE##Radius:)]) { \
|
|
|
|
view.border##SIDE##Radius = json ? [RCTConvert CGFloat:json] : defaultView.border##SIDE##Radius; \
|
|
|
|
} \
|
|
|
|
}
|
2015-05-13 18:22:21 +03:00
|
|
|
|
|
|
|
RCT_VIEW_BORDER_RADIUS_PROPERTY(TopLeft)
|
|
|
|
RCT_VIEW_BORDER_RADIUS_PROPERTY(TopRight)
|
2017-10-19 05:29:42 +03:00
|
|
|
RCT_VIEW_BORDER_RADIUS_PROPERTY(TopStart)
|
|
|
|
RCT_VIEW_BORDER_RADIUS_PROPERTY(TopEnd)
|
2015-05-13 18:22:21 +03:00
|
|
|
RCT_VIEW_BORDER_RADIUS_PROPERTY(BottomLeft)
|
|
|
|
RCT_VIEW_BORDER_RADIUS_PROPERTY(BottomRight)
|
2017-10-19 05:29:42 +03:00
|
|
|
RCT_VIEW_BORDER_RADIUS_PROPERTY(BottomStart)
|
|
|
|
RCT_VIEW_BORDER_RADIUS_PROPERTY(BottomEnd)
|
2015-05-13 18:22:21 +03:00
|
|
|
|
2017-06-21 03:12:52 +03:00
|
|
|
RCT_REMAP_VIEW_PROPERTY(display, reactDisplay, YGDisplay)
|
2016-06-09 19:48:56 +03:00
|
|
|
RCT_REMAP_VIEW_PROPERTY(zIndex, reactZIndex, NSInteger)
|
Implement CSS z-index for iOS
Summary:
This diff implement the CSS z-index for React Native iOS views. We've had numerous pull request for this feature, but they've all attempted to use the `layer.zPosition` property, which is problematic for two reasons:
1. zPosition only affects rendering order, not event processing order. Views with a higher zPosition will appear in front of others in the hierarchy, but won't be the first to receive touch events, and may be blocked by views that are visually behind them.
2. when using a perspective transform matrix, views with a nonzero zPosition will be rendered in a different position due to parallax, which probably isn't desirable.
See https://github.com/facebook/react-native/pull/7825 for further discussion of this problem.
So instead of using `layer.zPosition`, I've implemented this by actually adjusting the order of the subviews within their parent based on the zIndex. This can't be done on the JS side because it would affect layout, which is order-dependent, so I'm doing it inside the view itself.
It works as follows:
1. The `reactSubviews` array is set, whose order matches the order of the JS components and shadowView components, as specified by the UIManager.
2. `didUpdateReactSubviews` is called, which in turn calls `sortedSubviews` (which lazily generates a sorted array of `reactSubviews` by zIndex) and inserts the result into the view.
3. If a subview is added or removed, or the zIndex of any subview is changed, the previous `sortedSubviews` array is cleared and `didUpdateReactSubviews` is called again.
To demonstrate it working, I've modified the UIExplorer example from https://github.com/facebook/react-native/pull/7825
Reviewed By: javache
Differential Revision: D3365717
fbshipit-source-id: b34aa8bfad577bce023f8af5414f9b974aafd8aa
2016-06-07 17:40:25 +03:00
|
|
|
|
2015-03-17 17:04:39 +03:00
|
|
|
#pragma mark - ShadowView properties
|
2015-02-20 07:10:52 +03:00
|
|
|
|
2017-01-11 14:58:03 +03:00
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(top, YGValue)
|
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(right, YGValue)
|
2017-10-19 05:29:40 +03:00
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(start, YGValue)
|
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(end, YGValue)
|
2017-01-11 14:58:03 +03:00
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(bottom, YGValue)
|
2017-10-19 05:29:40 +03:00
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(left, YGValue)
|
2016-10-26 23:12:26 +03:00
|
|
|
|
2017-01-11 14:58:03 +03:00
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(width, YGValue)
|
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(height, YGValue)
|
2016-10-26 23:12:26 +03:00
|
|
|
|
2017-01-11 14:58:03 +03:00
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(minWidth, YGValue)
|
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(maxWidth, YGValue)
|
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(minHeight, YGValue)
|
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(maxHeight, YGValue)
|
2016-10-26 23:12:26 +03:00
|
|
|
|
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(borderTopWidth, float)
|
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(borderRightWidth, float)
|
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(borderBottomWidth, float)
|
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(borderLeftWidth, float)
|
2017-10-19 05:29:42 +03:00
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(borderStartWidth, float)
|
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(borderEndWidth, float)
|
2016-10-26 23:12:26 +03:00
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(borderWidth, float)
|
|
|
|
|
2017-01-11 14:58:03 +03:00
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(marginTop, YGValue)
|
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(marginRight, YGValue)
|
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(marginBottom, YGValue)
|
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(marginLeft, YGValue)
|
2017-10-19 05:29:46 +03:00
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(marginStart, YGValue)
|
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(marginEnd, YGValue)
|
2017-01-11 14:58:03 +03:00
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(marginVertical, YGValue)
|
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(marginHorizontal, YGValue)
|
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(margin, YGValue)
|
|
|
|
|
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(paddingTop, YGValue)
|
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(paddingRight, YGValue)
|
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(paddingBottom, YGValue)
|
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(paddingLeft, YGValue)
|
2017-10-19 05:29:44 +03:00
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(paddingStart, YGValue)
|
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(paddingEnd, YGValue)
|
2017-01-11 14:58:03 +03:00
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(paddingVertical, YGValue)
|
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(paddingHorizontal, YGValue)
|
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(padding, YGValue)
|
2016-10-26 23:12:26 +03:00
|
|
|
|
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(flex, float)
|
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(flexGrow, float)
|
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(flexShrink, float)
|
2017-01-11 14:58:03 +03:00
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(flexBasis, YGValue)
|
2016-12-02 16:47:43 +03:00
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(flexDirection, YGFlexDirection)
|
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(flexWrap, YGWrap)
|
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(justifyContent, YGJustify)
|
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(alignItems, YGAlign)
|
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(alignSelf, YGAlign)
|
Expose alignContent to react native
Summary:
This diff adds alignContent (https://developer.mozilla.org/en-US/docs/Web/CSS/align-content) support to React Native. This enables aligning the lines of multi-line content. See below playground for example usage.
```
class Playground extends React.Component {
render() {
return (
<View style={{width: '100%', height: '100%', flexDirection: 'row', backgroundColor: 'white', flexWrap: 'wrap', alignContent: 'space-between'}}>
<View style={{width: 100, height: 100, marginLeft: 10, marginTop: 10, backgroundColor: 'red'}}/>
<View style={{width: 100, height: 100, marginLeft: 10, marginTop: 10, backgroundColor: 'red'}}/>
<View style={{width: 100, height: 100, marginLeft: 10, marginTop: 10, backgroundColor: 'red'}}/>
<View style={{width: 100, height: 100, marginLeft: 10, marginTop: 10, backgroundColor: 'red'}}/>
<View style={{width: 100, height: 100, marginLeft: 10, marginTop: 10, backgroundColor: 'red'}}/>
<View style={{width: 100, height: 100, marginLeft: 10, marginTop: 10, backgroundColor: 'red'}}/>
<View style={{width: 100, height: 100, marginLeft: 10, marginTop: 10, backgroundColor: 'red'}}/>
</View>
);
}
}
```
Reviewed By: astreet
Differential Revision: D4611803
fbshipit-source-id: ae7f6b4b7e9f4bc78d2502da948214294aad4dd2
2017-03-01 20:12:48 +03:00
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(alignContent, YGAlign)
|
2016-12-02 16:47:43 +03:00
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(position, YGPositionType)
|
2016-11-23 18:32:08 +03:00
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(aspectRatio, float)
|
2022-10-21 00:53:32 +03:00
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(rowGap, float)
|
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(columnGap, float)
|
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(gap, float)
|
2015-03-26 07:29:28 +03:00
|
|
|
|
2016-12-02 16:47:43 +03:00
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(overflow, YGOverflow)
|
2017-03-01 20:12:50 +03:00
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(display, YGDisplay)
|
2016-08-30 10:58:15 +03:00
|
|
|
|
Added mechanism for directly mapping JS event handlers to blocks
Summary:
Currently, the system for mapping JS event handlers to blocks is quite clean on the JS side, but is clunky on the native side. The event property is passed as a boolean, which can then be checked by the native side, and if true, the native side is supposed to send an event via the event dispatcher.
This diff adds the facility to declare the property as a block instead. This means that the event side can simply call the block, and it will automatically send the event. Because the blocks for bubbling and direct events are named differently, we can also use this to generate the event registration data and get rid of the arrays of event names.
The name of the event is inferred from the property name, which means that the property for an event called "load" must be called `onLoad` or the mapping won't work. This can be optionally remapped to a different property name on the view itself if necessary, e.g.
RCT_REMAP_VIEW_PROPERTY(onLoad, loadEventBlock, RCTDirectEventBlock)
If you don't want to use this mechanism then for now it is still possible to declare the property as a BOOL instead and use the old mechanism (this approach is now deprecated however, and may eventually be removed altogether).
2015-09-02 15:58:10 +03:00
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(onLayout, RCTDirectEventBlock)
|
[ReactNative] Introduce onLayout events
Summary:
Simply add an `onLayout` callback to a native view component, and the callback
will be invoked with the current layout information when the view is mounted and
whenever the layout changes.
The only limitation is that scroll position and other stuff the layout system
isn't aware of is not taken into account. This is because onLayout events
wouldn't be triggered for these changes and if they are desired they should be
tracked separately (e.g. with `onScroll`) and combined.
Also fixes some bugs with LayoutAnimation callbacks.
@public
Test Plan:
- Run new LayoutEventsExample in UIExplorer and see it work correctly.
- New integration test passes internally (IntegrationTest project seems busted).
- New jest test case passes.
{F22318433}
```
2015-05-06 15:45:05.848 [info][tid:com.facebook.React.JavaScript] "Running application "UIExplorerApp" with appParams: {"rootTag":1,"initialProps":{}}. __DEV__ === true, development-level warning are ON, performance optimizations are OFF"
2015-05-06 15:45:05.881 [info][tid:com.facebook.React.JavaScript] "received text layout event
", {"target":27,"layout":{"y":123,"x":12.5,"width":140.5,"height":18}}
2015-05-06 15:45:05.882 [info][tid:com.facebook.React.JavaScript] "received image layout event
", {"target":23,"layout":{"y":12.5,"x":122,"width":50,"height":50}}
2015-05-06 15:45:05.883 [info][tid:com.facebook.React.JavaScript] "received view layout event
", {"target":22,"layout":{"y":70.5,"x":20,"width":294,"height":204}}
2015-05-06 15:45:05.897 [info][tid:com.facebook.React.JavaScript] "received text layout event
", {"target":27,"layout":{"y":206.5,"x":12.5,"width":140.5,"height":18}}
2015-05-06 15:45:05.897 [info][tid:com.facebook.React.JavaScript] "received view layout event
", {"target":22,"layout":{"y":70.5,"x":20,"width":294,"height":287.5}}
2015-05-06 15:45:09.847 [info][tid:com.facebook.React.JavaScript] "layout animation done."
2015-05-06 15:45:09.847 [info][tid:com.facebook.React.JavaScript] "received image layout event
", {"target":23,"layout":{"y":12.5,"x":82,"width":50,"height":50}}
2015-05-06 15:45:09.848 [info][tid:com.facebook.React.JavaScript] "received view layout event
", {"target":22,"layout":{"y":110.5,"x":60,"width":214,"height":287.5}}
2015-05-06 15:45:09.862 [info][tid:com.facebook.React.JavaScript] "received text layout event
", {"target":27,"layout":{"y":206.5,"x":12.5,"width":120,"height":68}}
2015-05-06 15:45:09.863 [info][tid:com.facebook.React.JavaScript] "received image layout event
", {"target":23,"layout":{"y":12.5,"x":55,"width":50,"height":50}}
2015-05-06 15:45:09.863 [info][tid:com.facebook.React.JavaScript] "received view layout event
", {"target":22,"layout":{"y":128,"x":60,"width":160,"height":337.5}}
```
2015-05-07 22:11:02 +03:00
|
|
|
|
2017-02-07 07:58:23 +03:00
|
|
|
RCT_EXPORT_SHADOW_PROPERTY(direction, YGDirection)
|
Implement CSS z-index for iOS
Summary:
This diff implement the CSS z-index for React Native iOS views. We've had numerous pull request for this feature, but they've all attempted to use the `layer.zPosition` property, which is problematic for two reasons:
1. zPosition only affects rendering order, not event processing order. Views with a higher zPosition will appear in front of others in the hierarchy, but won't be the first to receive touch events, and may be blocked by views that are visually behind them.
2. when using a perspective transform matrix, views with a nonzero zPosition will be rendered in a different position due to parallax, which probably isn't desirable.
See https://github.com/facebook/react-native/pull/7825 for further discussion of this problem.
So instead of using `layer.zPosition`, I've implemented this by actually adjusting the order of the subviews within their parent based on the zIndex. This can't be done on the JS side because it would affect layout, which is order-dependent, so I'm doing it inside the view itself.
It works as follows:
1. The `reactSubviews` array is set, whose order matches the order of the JS components and shadowView components, as specified by the UIManager.
2. `didUpdateReactSubviews` is called, which in turn calls `sortedSubviews` (which lazily generates a sorted array of `reactSubviews` by zIndex) and inserts the result into the view.
3. If a subview is added or removed, or the zIndex of any subview is changed, the previous `sortedSubviews` array is cleared and `didUpdateReactSubviews` is called again.
To demonstrate it working, I've modified the UIExplorer example from https://github.com/facebook/react-native/pull/7825
Reviewed By: javache
Differential Revision: D3365717
fbshipit-source-id: b34aa8bfad577bce023f8af5414f9b974aafd8aa
2016-06-07 17:40:25 +03:00
|
|
|
|
2022-02-10 17:05:21 +03:00
|
|
|
// The events below define the properties that are not used by native directly, but required in the view config for new
|
|
|
|
// renderer to function.
|
|
|
|
// They can be deleted after Static View Configs are rolled out.
|
|
|
|
|
|
|
|
// PanResponder handlers
|
|
|
|
RCT_CUSTOM_VIEW_PROPERTY(onMoveShouldSetResponder, BOOL, RCTView) {}
|
|
|
|
RCT_CUSTOM_VIEW_PROPERTY(onMoveShouldSetResponderCapture, BOOL, RCTView) {}
|
|
|
|
RCT_CUSTOM_VIEW_PROPERTY(onStartShouldSetResponder, BOOL, RCTView) {}
|
|
|
|
RCT_CUSTOM_VIEW_PROPERTY(onStartShouldSetResponderCapture, BOOL, RCTView) {}
|
|
|
|
RCT_CUSTOM_VIEW_PROPERTY(onResponderGrant, BOOL, RCTView) {}
|
|
|
|
RCT_CUSTOM_VIEW_PROPERTY(onResponderReject, BOOL, RCTView) {}
|
|
|
|
RCT_CUSTOM_VIEW_PROPERTY(onResponderStart, BOOL, RCTView) {}
|
|
|
|
RCT_CUSTOM_VIEW_PROPERTY(onResponderEnd, BOOL, RCTView) {}
|
|
|
|
RCT_CUSTOM_VIEW_PROPERTY(onResponderRelease, BOOL, RCTView) {}
|
|
|
|
RCT_CUSTOM_VIEW_PROPERTY(onResponderMove, BOOL, RCTView) {}
|
|
|
|
RCT_CUSTOM_VIEW_PROPERTY(onResponderTerminate, BOOL, RCTView) {}
|
|
|
|
RCT_CUSTOM_VIEW_PROPERTY(onResponderTerminationRequest, BOOL, RCTView) {}
|
|
|
|
RCT_CUSTOM_VIEW_PROPERTY(onShouldBlockNativeResponder, BOOL, RCTView) {}
|
|
|
|
|
|
|
|
// Touch events
|
|
|
|
RCT_CUSTOM_VIEW_PROPERTY(onTouchStart, BOOL, RCTView) {}
|
|
|
|
RCT_CUSTOM_VIEW_PROPERTY(onTouchMove, BOOL, RCTView) {}
|
|
|
|
RCT_CUSTOM_VIEW_PROPERTY(onTouchEnd, BOOL, RCTView) {}
|
|
|
|
RCT_CUSTOM_VIEW_PROPERTY(onTouchCancel, BOOL, RCTView) {}
|
|
|
|
|
2022-04-19 01:47:55 +03:00
|
|
|
// Experimental/WIP Pointer Events (not yet ready for use)
|
2022-04-08 00:07:58 +03:00
|
|
|
RCT_EXPORT_VIEW_PROPERTY(onPointerCancel, RCTBubblingEventBlock)
|
|
|
|
RCT_EXPORT_VIEW_PROPERTY(onPointerDown, RCTBubblingEventBlock)
|
2022-07-06 06:00:42 +03:00
|
|
|
RCT_EXPORT_VIEW_PROPERTY(onPointerMove, RCTBubblingEventBlock)
|
2022-04-08 00:07:58 +03:00
|
|
|
RCT_EXPORT_VIEW_PROPERTY(onPointerUp, RCTBubblingEventBlock)
|
2022-07-06 06:00:42 +03:00
|
|
|
RCT_EXPORT_VIEW_PROPERTY(onPointerEnter, RCTCapturingEventBlock)
|
|
|
|
RCT_EXPORT_VIEW_PROPERTY(onPointerLeave, RCTCapturingEventBlock)
|
2022-06-08 02:08:50 +03:00
|
|
|
RCT_EXPORT_VIEW_PROPERTY(onPointerOver, RCTBubblingEventBlock)
|
|
|
|
RCT_EXPORT_VIEW_PROPERTY(onPointerOut, RCTBubblingEventBlock)
|
2022-04-08 00:07:58 +03:00
|
|
|
|
2015-02-20 07:10:52 +03:00
|
|
|
@end
|